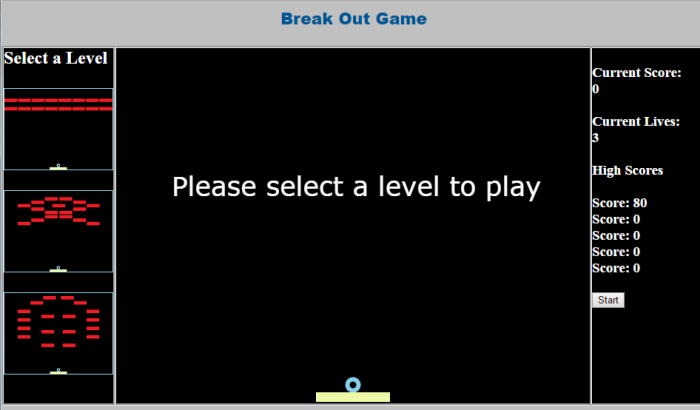
The Breakout game featured three different levels. This was done by creating the bricks for all three levels, and then depending on which level was selected the game would add the bricks for that level into the game. To select the level each of the three images at the left side of the screen could be interacted with, each image has a onclick feature which will call the function “ChooseLevelOne()”, “ChooseLevelTwo()” or “ChooseLevelThree()” depending on which image was pressed, this would then assign the variable “Level” to the appropriate number and the functions “restart()”, “stopgame()” and “removeblocks()” would be called to reset the ball and paddle variables and clear the screen of remaining blocks.
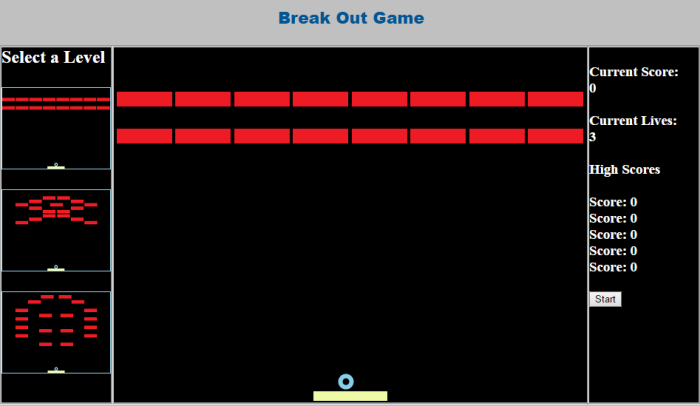
This is a screenshot of level one from my breakout game. It features two lines of blocks for the player to destroy. When all of these blocks have been destroyed the level has been complete and the game will return to the level selection screen.
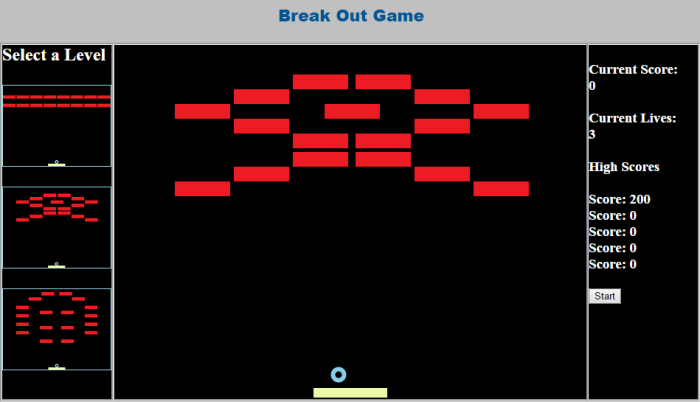
This is a screenshot of level two from my breakout game. It features a formation of seventeen blocks. When all of these blocks have been destroyed the level has been complete and the game will return to the level selection screen.
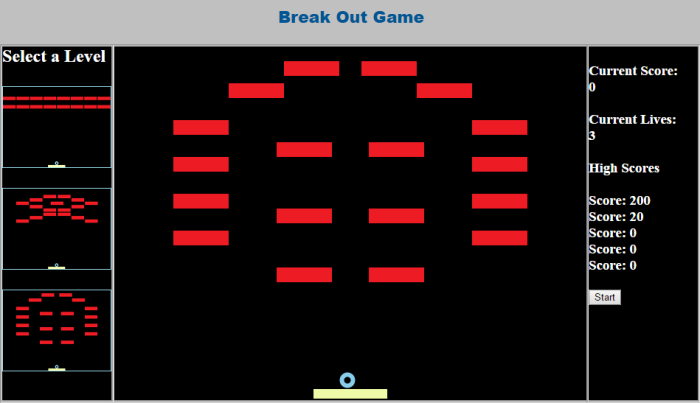
This is a screenshot of level three from my breakout game. It features a formation of eighteen blocks. When all of these blocks have been destroyed the level has been complete and the game will return to the level selection screen.
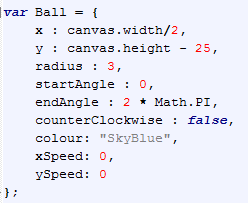
The ball is created using as constructor that assigns the position, colour, speed, radius and start and end angle.

The balls movement was done with two simple lines of code which was took the x and y speed of the ball and took away the value of x speed and y speed every second to change the balls position.
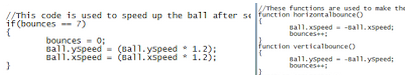
The ball has a variable called “bounces”, everytime the ball bounces off a brick or the paddle this variable is incremented. When “bounces” is equal to 7 it is reset to 0 and the x and y speed of the ball is increased by 20%.
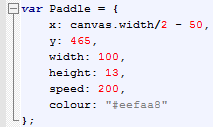
The paddle was created using a constructor which sets the position, size, speed and colour of the paddle.
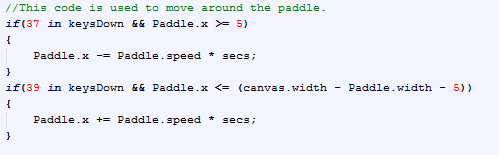
To move the paddle around the player must use the left and right arrow keys. This is implemented into the code with two if statements which increase or decrease the paddles x position depending on which arrow key is pressed.
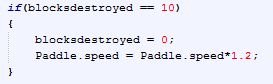
Every time that a block is destroyed the variable “blocksdestroyed” is incremented, when this value is equal to 10 it gets reset and the speed of the paddle is increased by 20%.
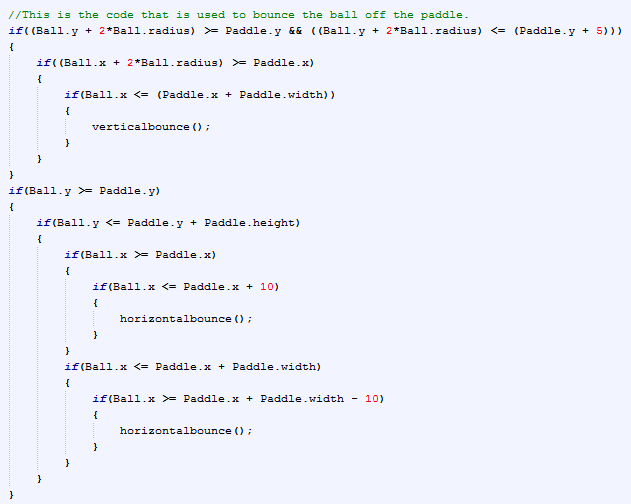
If the ball collides with the paddle horizontally then the x speed of the ball is inverted and the variable “bounces” is incremented. If the ball collides with the paddle vertically then the y speed of the ball is inverted and the variable “bounces” is incremented.
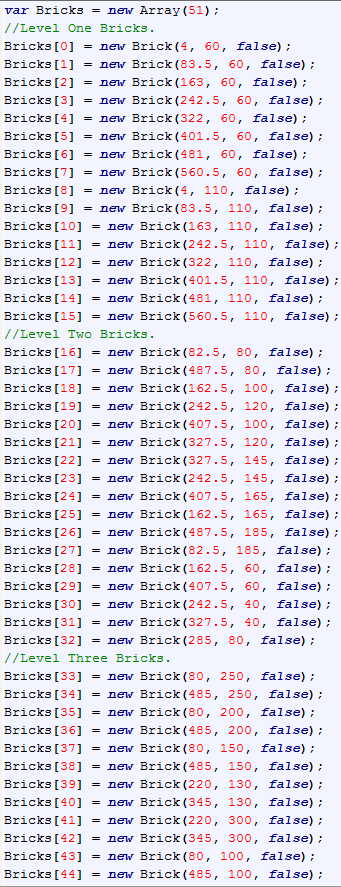
To create the bricks an array of 51 values was created, which would store two integers and a boolean. Each value in the array was then given the values of each brick from each level. All of the bricks were set to invisible as they are only able to be interacted with when they are visible. Each brick would create a new “Brick” which was a function that set the x, y and visibility of the brick to the value given when the brick was declared.
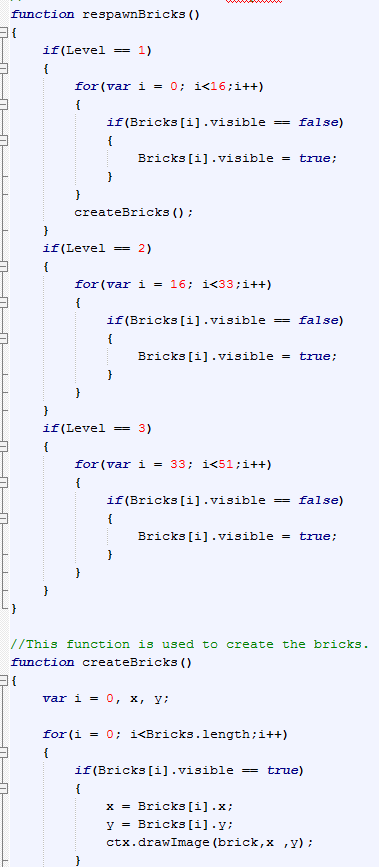
When a level is started all of the bricks for that level are set to visible. The function “createBricks()” is then used to draw every brick which is visible, using the x and y values to position the bricks and using the variable “brick” (which is storing the brick image) to draw the image from that point.
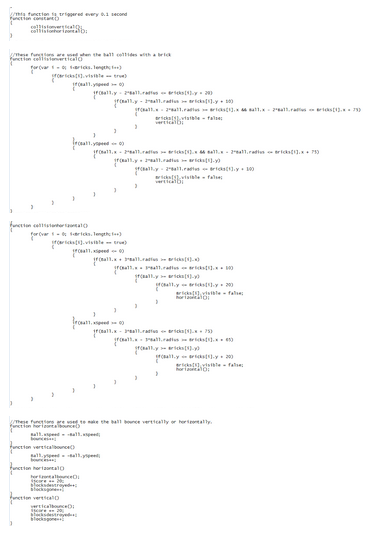
Every second the program tests to see if the ball has collided with a brick, and if so whether it is a vertical or horizontal collision. If there is a collision then the brick the ball collides with is made invisible. If it is a vertical collision the function “vertical()” is called, if the collision is horizontal the function “horizontal()” is called. Both functions are similar as they increase the value of the variable “iScore” by 20 and increments the variables “blocksdestroyed” and “blocksgone”. The function “vertical()” calls the function “verticalbounce()”, which inverts the y speed of the ball and increments the variable “bounces”, whereas the function “horizontal()” calls the function “horizontalbounce()”, which inverts the x speed of the ball and increments the variable “bounces”.
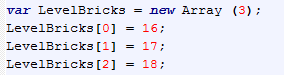
Before starting to code the levels each level was planned out, this was so that the bricks would be in the correct position without needing to use trial and error to place them in the correct position. Each brick was then individually given an x and y value of where to be drawn. An array of 3 values was created to store how many bricks were to be in each level.
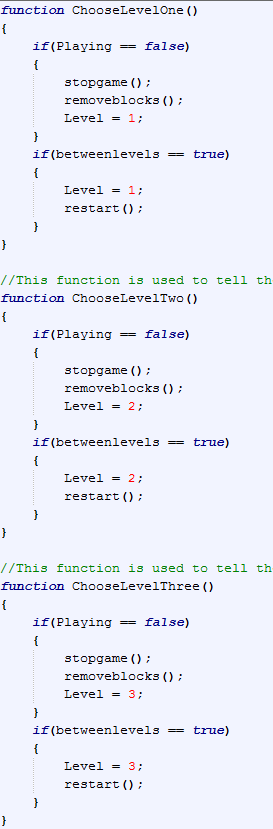
To choose the level the player wishes to play, the player must click on the image of the level and press start. This will then change the variable “Level” to either 1, 2 or 3 depending on the desired level.
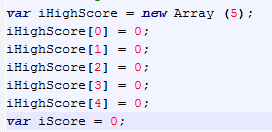
The score is represented as both the integer “iScore”, which is used during the game, and the array “iHighScore[5]”, which has five values used to show the high scores.
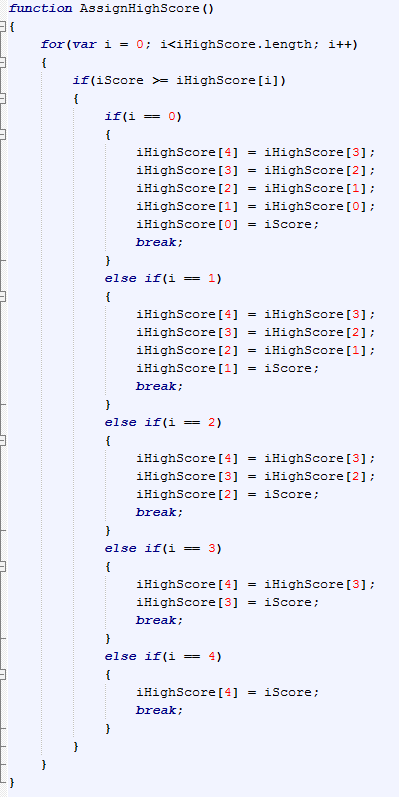
When the player runs out of lives and the game is over the functions “AssignHighScore()”, “SetHighScore()” and “stopgame()” are called. “AssignHighScore()” goes through each previous high score to see if the value if “iScore” is higher than any of them, if “iScore” is higher than the value it replaces it and all high scores lower are assigned to the high score below it.
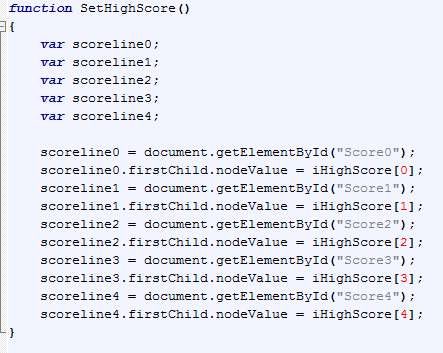
The function “SetHighScore()” is used to change the text on screen, that displays the high scores, to display the new high score values if they have changed at all.
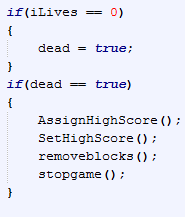
When the variable “iLives” is equal to 0 then the game is over, the boolean “dead” is set to true and the game calls the functions “AssignHighScore()”, “SetHighScore()”, “removeblocks()” and “stopgame()”.
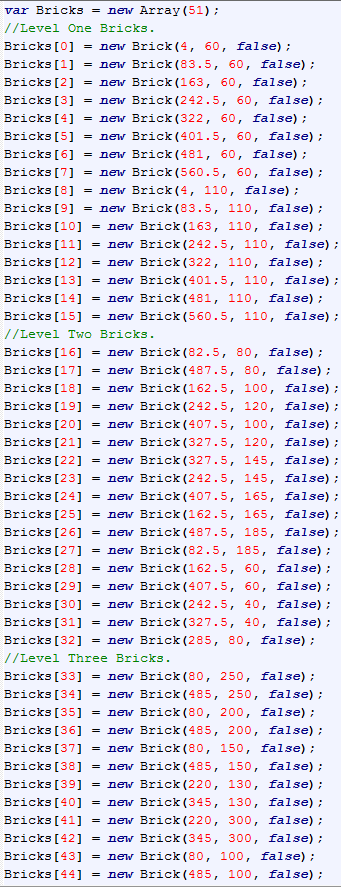
If this project was repeated the way that the bricks were constructed would be different. The array of bricks would only be the size of the most bricks in each level. Each brick would then be given the x and y values for each level rather than just one level, if the brick is not to be displayed on a certain level the values given would be 0. There would then be an if statement which would set the visibility of the brick to false if the values of both x and y are equal to 0.
E.g. Brick one is to be displayed on level one, two and three.
Bricks[1] = new Brick(4, 60, 50, 100, 70, 80)
On level one it would be displayed at x 4 and y 60, on level two it would be displayed at x 50 and y 100 and on level three it would be displayed at x 70 and y 80.
On the other hand brick five is only displayed on level two.
Bricks[5] = new Brick (0, 0, 100, 60, 0, 0)
On level two the brick would be placed in the position x 100, y 60 but on the other two levels the brick would not be drawn onto the screen.
gLike